Wat is legacy?
Laten we beginnen met een definitie. Want wanneer spreken we nu van Legacy in IT? We zijn het eens met Wikipedia, daar zeggen ze (vrij vertaald) het volgende. Bij Legacy-systemen hebben we het over een āāmethode, technologie, systeem of applicatie gekoppeld aan ā of onderdeel van ā een verouderd systeem. Aan deze software wordt niet meer doorontwikkelt, meestal omdat ze niet meer aan de huidige, moderne standaard en/of eisen voldoen. Maar ook onderhoud en ondersteuning wordt vaak complexer en duurder in de loop der jaren. En dat kan natuurlijk best wel een gevaar betekenen voor jouw business.
Er bestaat ook Legacy-code. Dat is broncode die niet langer (goed) wordt ondersteund en die doorontwikkeling, maar ook onderhoud, eraan lastig en intensief maakt.
EOL oftewel End of Life
Alle software heeft een bepaalde levensduur. Het begint met een lancering. Dan volgt een periode van onderhoud, doorontwikkeling en dan komt daar de aankondiging ā meestal na jaren ā dat het pakket of product zijn End of Life nadert. Kort na zoān aankondiging zal het product niet langer actief verkocht worden. En daarna koerst de leverancier af op een datum waarop zij niet langer verantwoordelijk zijn voor de software. Vanaf dat moment gebruik je deze software dus op eigen risico!
Dat hoeft overigens niet direct erg te zijn. Vaak kunnen legacy systemen nog jaren mee. Mits ze voldoen aan moderne veiligheidseisen en ze te koppelen zijn met moderne systemen. Check vooral ook of je hoofdontwikkelaar nog niet met pensioen gaat. š Je zou zelfs kunnen zeggen dat het voordeel van legacay is dat de kinderziekten en bugs er vaak allang uit zijn. Het kan dan ook als betrouwbaarder en stabieler worden ervaren dan wanneer je āmoetā pionieren met āhet nieuwste van het nieuwsteā.
Maar dan komt er toch altijd een moment dat je er niet langer onderuit kunt. Je systemen zijn echt toe aan vervanging.
3 grootste problemen van legacy
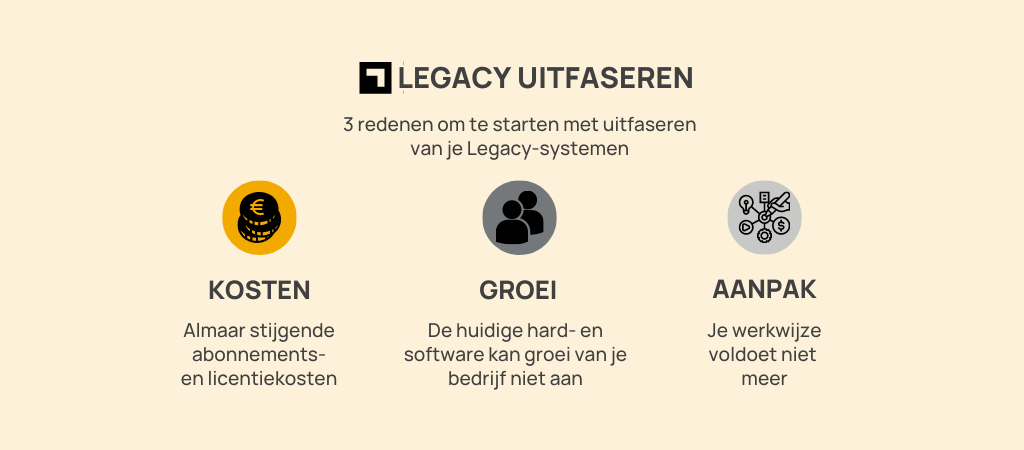
- Kosten
De kans is groot dat je te maken hebt met hoge licentiekosten. Door over te stappen op een nieuw pakket of nieuwe tool kun je vaak snelle euroās besparen. Omdat je ze niet langer zomaar uitgeeft aan licenties die niet langer bij je passen. - Groei
Je wilt vernieuwen als organisatie, maar de oude software staat die vernieuwing in de weg. In de afgelopen jaren heb je zoveel snelle fixes en verbeteringen doorgevoerd (shortcuts), dat het door de ingewikkelde structuren moeilijk is geworden om nieuwe features te ontwikkelen binnen dezelfde code. - Aanpak
Je wilt bijvoorbeeld realtime data ontsluiten via een app, maar je huidige IT vormt daarin een belemmering. Het zijn die momenten waarop je flexibiliteit nodig hebt, maar vastzit aan oude software of een oud pakket. Automatiseren van bedrijfsprocessen wordt ook een heuse uitdaging met Legacy en je zult langzaamaan slagvaardigheid en efficiency verliezen.
Uitfaseren, neem de tijd
Transformeren van legacy-systemen naar flexibele en snel innoverende software doe je niet in een dag. Je hebt te maken met een aantal, grote uitdagingen:
- Software-ontwikkeling is complex
- Je bestaande IT-infrastructuur is niet gebouwd op verandering en innovatie, maar op stabiliteit en continuĆÆteit
- Huidige IT-systemen zijn niet flexibel en vaak georganiseerd in siloās, waarin website, webshop, CRM, etc. niet gemaakt zijn om gegevens uit te wisselen.Ā
Big bang versus uitfaseren
Het vernieuwen van software en systemen is een flinke investering, vooral als je complete IT-architectuur op de schop moet. Je moet immers elk aspect herontwerpen, herbouwen, herinrichten en opnieuw leren in gebruik te nemen. Dat kost veel tijd van je mensen en vraagt veel van je IT-budget. Hoe groter en complexer je systeem en infrastructuur, hoe langer het vernieuwingstraject duurt, al pak je het nog zoĀ agileĀ aan. Ga je eenmaal met een ābig bangā na maanden ontwikkelen live, dan begint het pas. Je bent niet klaar met software, je zult blijvend moeten verbeteren en doorontwikkelen om te voorkomen dat ook je nieuwe systemen legacy-systemen wordenā¦
Het is onze ervaring dat een livegang met een big bang niet meer van nu is. Je gaat niet maanden of jaren ontwikkelen om op een magisch moment je software officieel in gebruik te nemen en er dan achter te komen wat nog mist. Of erger: dat je erachter komt wat je niet nodig had. We werken zelf al sinds 2011Ā 100% AgileĀ met onder andere Scrum en Kanban, dus het zit in onze aard om live te gaan zodra het kan. Vanaf dat moment bouw je verder uit met de feedback en ervaring van je gebruikers.Ā
Een Agile aanpak is er dus op gericht om gefaseerd te werken, klein te beginnen en dat uit te bouwen. Je kunt je legacy-systemen dus beterĀ uitfaseren. Waarbij je ze opdeelt in stukken (services of componenten) om die vervolgens stuk voor stuk te verbeteren, te herbouwen en te vervangen. Het voordeel is dat je in korte tijd al live kan met iets nieuws, terwijl je oude systemen gewoon actief blijven zolang dat nodig is.
Weet je niet zo goed waar je moet beginnen?
Als wij starten met een uitfaseringstraject, dan kijken we eerst naar wat er nu draait, wat daarin bedrijfskritisch is en hoe we systemen kunnen opdelen in hapklare brokken.Ā Om je een perfecte kickstart te geven kan het prettig zijn eerst die kennis op te doen. Over hoe je eigen platform precies in elkaar zit, en over waar je naartoe kunt. Daarom kun je bij ons een Replatforming Workshop volgen. We organiseren hem regelmatig en deelname is gratis! Gewoon, omdat we dol zijn op kennis delen. Meld je aan voor de gratis Workshop Replatforming