Now that React 16 is finally released ? I thought it would be time for a nice article (or maybe a serie of articles ?) about React and its ecosystem! This article is meant as a nice introduction to React and the ecosystem. In future publications I would like to go more in depth about performance improvements React 16 provides.
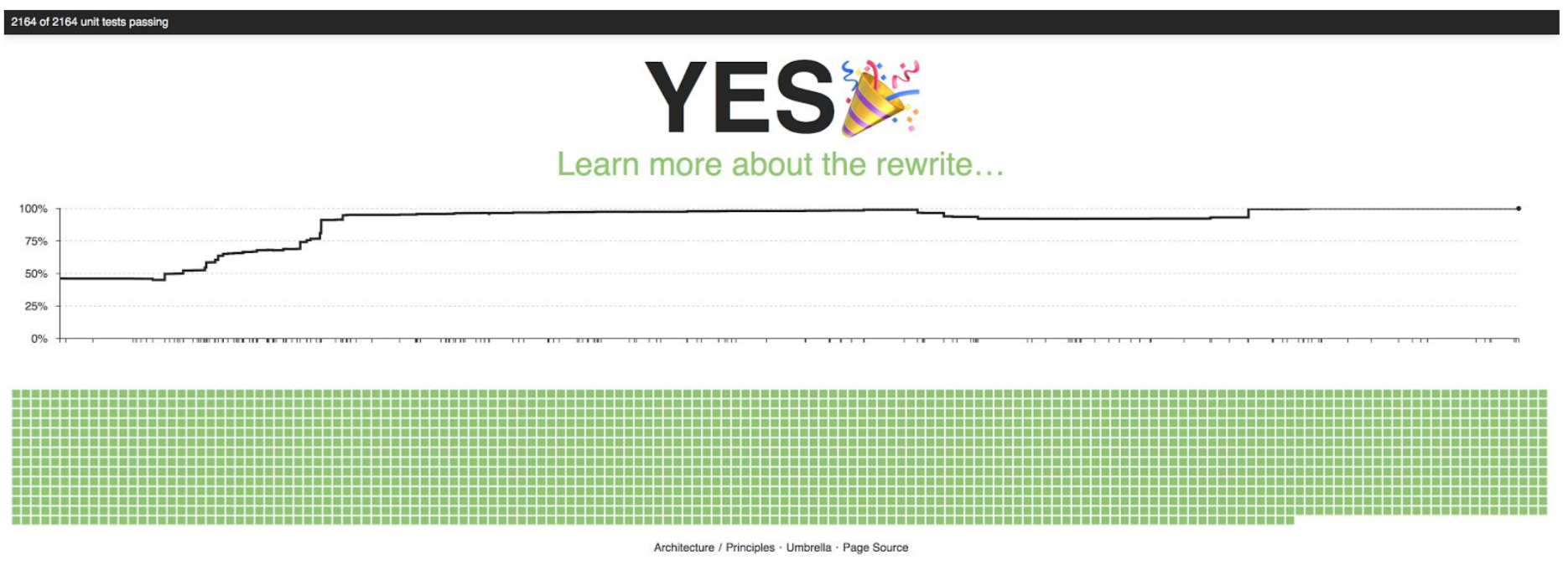
Not a tool or language, it is a (view) framework
React is a JavaScript framework which abstracts the view layer of an application.
When using React you split up your application in lots of smaller components. A component consists of event handling, styling and sometimes state. This makes a component an isolated and reusable piece. These components can be combined into larger components.
const Child = () => <div>{`i'm a child! \o/`}</div>
class Parent extends React.Component {
render() {
return (<div><Child /></div>);
}
}
A React component
Reacts markup language JSX
React components are written in a markup language called JSX (JavaScriptXML), an XML/HTML like structure. When you are a web developer and you use React for the web you’ll be familiar with most of the JSX component since they’re almost identical to plain HTML components.
By default the JSX code cannot be parsed by the JavaScript runtime. This is where transpilation comes in. To run the JSX code the code is first transpiled using Babel. This basically means to restructure the code from format A to format B. The code snippets below shows an example of simple JSX transpilation.
import React from 'react';
const HelloWorld = () => <div>Hello world!</div>
export default HelloWorld;
Code before Babel transpilation
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _react = require('react');
var _react2 = _interopRequireDefault(_react);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var HelloWorld = function HelloWorld() {
return _react2.default.createElement(
'div',
null,
'Hello world!'
);
};
exports.default = HelloWorld;
Code after Babel transpilation
As seen in the snippets al the JSX code is now transformed in React.createElement() calls.
Working with state
Every React component can contain state. In the most basic form state is just an object which lives inside a component. The idea is that every time the state within the component changes, the component will re-render using this new state. The diffing algorithm then decides which parts of the component needs updating. An example of state in a component can be a simple increment. Every time the setState is called the component will re-render. When your application grows larger and larger you might want to look into a more robust state management system such as Redux.
class Clock extends React.Component {
state = {
increment: 0,
};
componentDidMount() {
setInterval(() => {
this.setState({
increment: this.state.increment + 1,
})
}, 2000)
}
render() {
return (<div>{this.state.increment}</div>);
}
}
ReactDOM.render(
<Clock />,
document.getElementById('root')
);
State in a React component
Working with styling
A React Component can also contain styling. The most basic way of styling is filling the style property of a component. This property accepts an object of CSS rules in a key-value pair. You can also use a more classic way of styling by referring to style using the className property. The issue with this however is that you make it harder to reuse your components elsewhere. Using the style property of a component is a basic way of styling, there are tons of libraries which can make styling components easier.
const style = {
backgroundColor: 'green'
};
class Child = () => <div style={style}></div>
// or using styles
class Child = () => <div className='<yourClass>'></div>
A form of styling in a React component
Rendering with Virtual DOM
The React components are rendered in a thing called the Virtual DOM. The virtual DOM is a browser independent implementation of the DOM and does smart stuff like batching before applying changes to the real DOM. The virtual DOM can then be applied to the underlying platform using tools such as React DOM. This operation saves the developer a lot of work and increases the rendering performance (in most cases). Also the developer doesn’t have to think about how and where a node should be placed.
Every component can represent a part of the view. These components together form a tree structure. React then decides when a change occurs what part of the tree should be re-rendered. The algorithm to decide if a component should be updated or not is called ‘Reconciliation‘.
React in itself cannot render into a browser or into any other type of application. To render this kind of application in the browser for example a tool is required called ‘React DOM’.  The main responsibility of React DOM is that your components will be rendered correctly into the browser dom. Other responsibilities of React DOM are basic event handling.
React can be used in much more places than just in the browser. In the next few sections I listed some alternative platforms where React can be used. These are only a few of many implementations.
Native
React native is React on mobile platforms (Android and iOS). Native uses the Native components of the platform on the device. Every component has a bridge which is written in native code. This bridge can communicate from JavaScript to the native iOS or Android components.
VR
Did you read this correctly?? Yes. React VR is React for Virtual Reality experiences. React VR uses WebVRÂ but it makes it easier to write VR applications using the well known React structure.
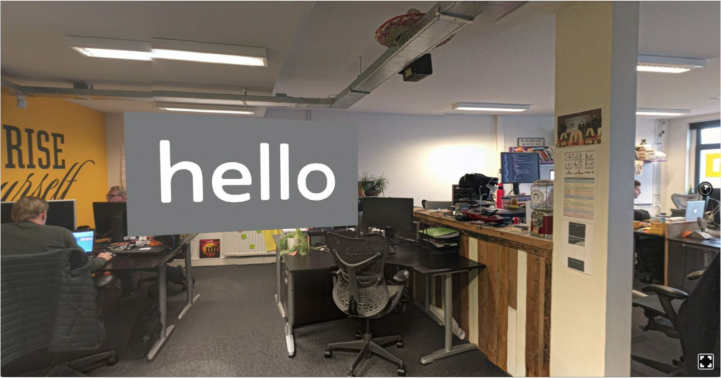
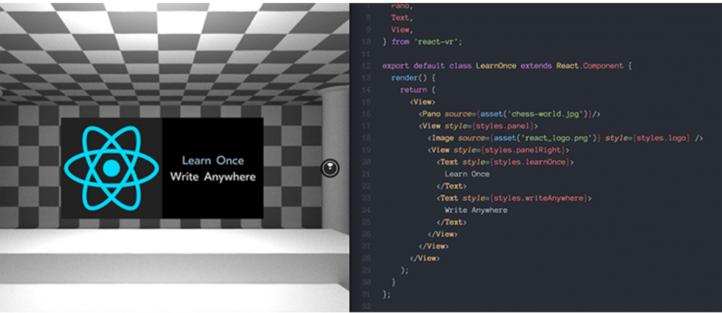
Blessed
React blessed is a library to render components in a terminal.Â
Native macOS
React Native macOS is like the ‘normal’ native, but then for the macOS platform.
Hardware
With React hardware (Arduino on steroids ?) you can talk to real hardware. For example to turn a Led on and off.
import React from 'react';
import ReactHardware, {Board, Led} from 'react-hardware';
const HIGH = 255;
const LOW = 0;
class FlashingLed extends React.Component {
constructor(props) {
super(props);
this.state = {value: 0};
this._timer = null;
this.defaultProps = {
interval: 1000,
};
}
componentDidMount() {
this._timer = setInterval(_ => (
this.setState(prevState => ({value: prevState.value === HIGH ? LOW : HIGH}))
), this.props.interval);
}
componentWillUnmount() {
clearInterval(this._timer);
this._timer = null;
}
render() {
return (
<Led {...this.props} value={this.state.value} />
);
}
}
class Application extends React.Component {
render() {
return (
<Board>
<FlashingLed pin={9} />
<FlashingLed pin={10} />
<FlashingLed pin={11} />
<FlashingLed pin={12} />
</Board>
);
}
}
var PORT = '/dev/tty.usbmodem1411';
ReactHardware.render(<Application />, PORT);
React in the hardware world
What problem doesn’t React solve?
React solves the view rendering part. Most applications are way more complex than just plain template rendering. It doesn’t provide solutions for things like data fetching, state management, the way you style your application or your overall application structure. This is something you’ll have to implement yourself using libraries such as Redux or Mobx (for state management). You can also use a more framework like implementation of React such as Next.js. So in order to use React in larger projects you have quite some boxes to check ✅.
Possible future articles ?
- Performance improvements in React 16
- Going fancy with styled components
- State management with Redux
- Flow as a static type checker
- Webpack module bundler
- Debugging React
- What is Babel and how does it work?